iOS 15 packed with cool features for developers like SharePlay Facetime Link and custom sized keyboard
Introduction
Did you watch WWDC June’21? It was packed with loads of exciting stuff for developers like iOS 15, iPadOS 15 (cousin of iOS đ±) FaceTime links, SharePlay, GroupActivities.
And an unbelievable feature from Disciplined Apple called resizing your keyboard, yes now you can change the size of the keyboard or move it around.
Let’s look at the top 5 features of iOS 15
- UISheetPresentationController
- UIKeyboardLayoutGuide
- ShazhamKit & MusicKit
- CoreLocation UI
- Group Activity & Share Play
UISheetPresentationController
Itâs just another way of presenting UIViewController, earlier you could present modally. If you donât remember here is a sample
let viewController = UIViewController()
viewController.modalPresentationStyle = .pageSheet
self.present(viewController, animated: true, completion: {})
Now with UISheetPresentationController you can present your View Controller in two sizes, medium height and full height.
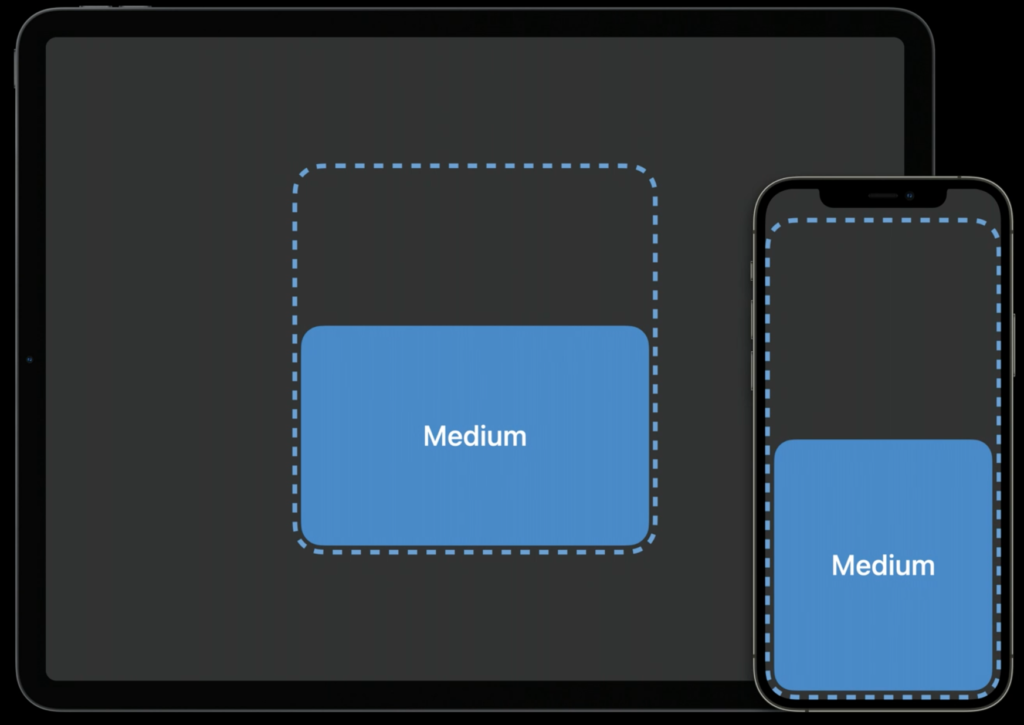
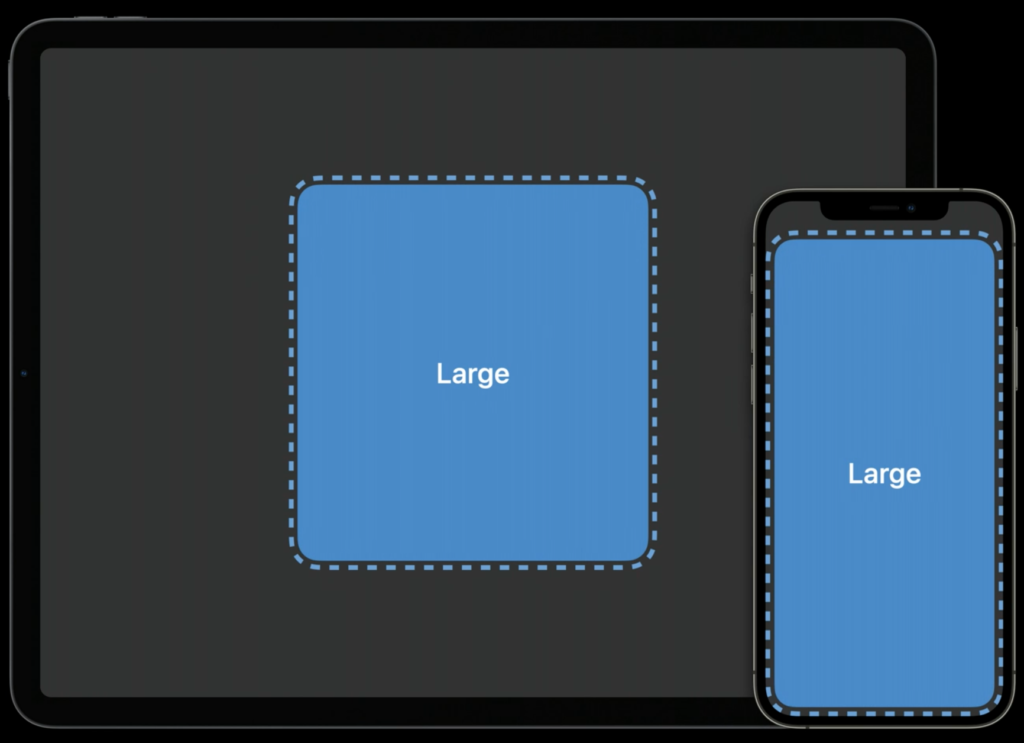
Look at the code how to present UISheetPresentationController
let viewController = UIViewController()
viewController.detents = [.medium()]
self.present(viewController, animated: true, completion: {})
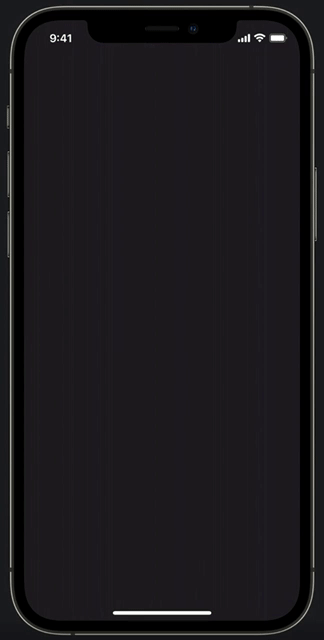
Interestingly you can present in both medium and large sizes as well, which means height of the view controller can be changed depending on what content is being shown in the UI.
let viewController = UIViewController()
viewController.detents = [.medium(), .large()]
self.present(viewController, animated: true, completion: {})
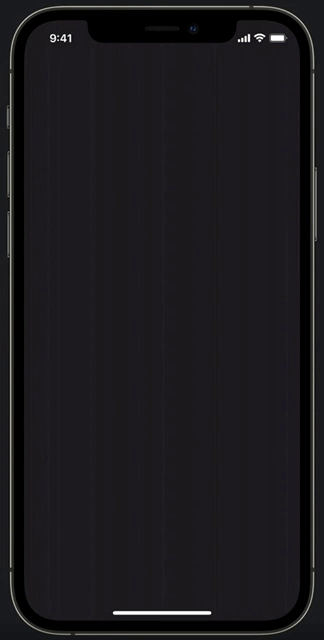
UIKeyboardLayoutGuide
Keyboard is all yours now, you can place wherever you like, under an ImageView above a TextField etc.. No Hassle of observing notification anymore
Keyboard used to always appear on the bottom isn’t it? Now it can be placed according to the need. Layout can be adjusted by setting top, bottom, leading and trailing anchors.
view.keyboardLayoutGuide.topAnchor.constraint(equalTo: textField.bottomAnchor).isActive = true
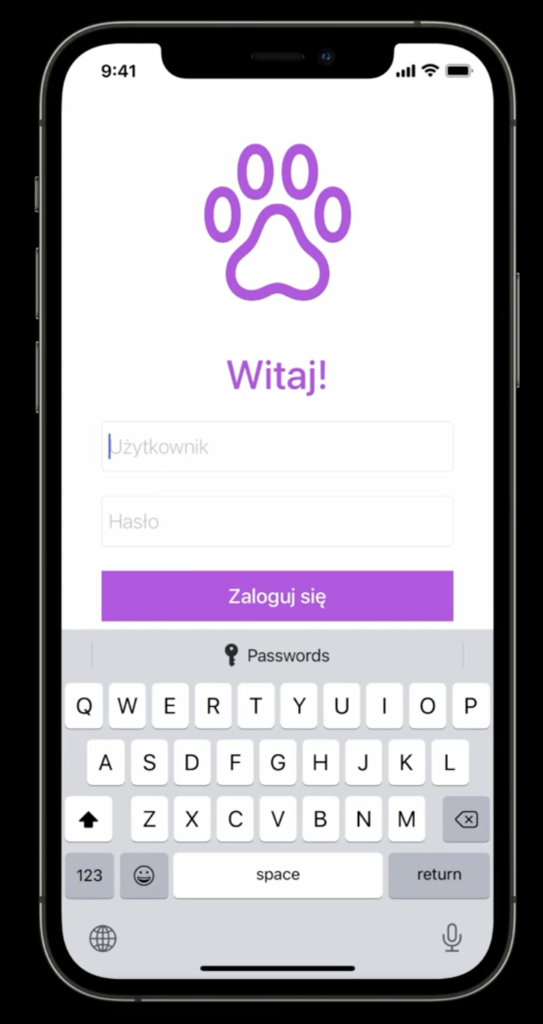
In case you need to take the keyboard to top or any other place you can use following code, but you need to define how the UI changes keyboard moves.
view.keyboardLayoutGuide.followsUndockedKeyboard = true
ShazhamKit
Have you ever faced that such situation that you don’t know the song but you know the tune?
All you need to do is hymn a tune or few words and Shazham app will find the real song. If you don’t know about Shazham app then go and search it in AppStore.
Also to tell you some history it was acquired by Apple in 2018 checkout this Techcrunch Article.
After 3 years of acquisition of Shazham, Apple decided to release it as service for iOS apps that can leverage this music finding feature with no extra cost. iOS 15 have this amazing feature named ShazhamKit.
Let’s see how we really integrate that using code snippet:
import UIKit
import AVFoundation
import ShazamKit
class HomeViewController: UIViewController, SHSessionDelegate {
var audioRecorder: AVAudioRecorder!
@IBAction func findSong() {
checkPermissionToRecord()
}
@IBAction func stopRecording() {
guard let audioURL = audioPath,
let file = try? AVAudioFile(forReading: audioURL),
let format = AVAudioFormat(commonFormat: .pcmFormatFloat32, sampleRate: file.fileFormat.sampleRate, channels: 1, interleaved: false),
let buffer = AVAudioPCMBuffer(pcmFormat: format, frameCapacity: 1024) else { return }
try? file.read(into: buffer)
findMatchingSong(buffer)
}
func checkPermissionToRecord() {
guard AVAudioSession.sharedInstance().recordPermission != .granted else { return }
AVAudioSession.sharedInstance().requestRecordPermission({ allowed in
if allowed {
self.recordAudio()
}
})
}
func recordAudio() {
guard let audioURL = audioPath else { return }
let audioSession:AVAudioSession = AVAudioSession.sharedInstance()
try? audioSession.setCategory(AVAudioSession.Category.playAndRecord)
try? audioSession.setActive(true, options: .notifyOthersOnDeactivation)
let recordSettings = [AVFormatIDKey:kAudioFormatAppleIMA4,
AVSampleRateKey:44100.0,
AVNumberOfChannelsKey:2,AVEncoderBitRateKey:12800,
AVLinearPCMBitDepthKey:16,
AVEncoderAudioQualityKey:AVAudioQuality.max.rawValue] as [String : Any]
print("Audio Recording Available at Path : \(audioURL)")
audioRecorder = try? AVAudioRecorder.init(url: audioURL, settings: recordSettings)
audioRecorder.record()
}
var audioPath : URL? {
guard let documentsDir = NSSearchPathForDirectoriesInDomains(FileManager.SearchPathDirectory.documentDirectory, FileManager.SearchPathDomainMask.userDomainMask, true).first else { return .none }
return URL.init(fileURLWithPath: documentsDir + "/recordTest.mp3")
}
func findMatchingSong(_ audioBuffer: AVAudioPCMBuffer) {
let session = SHSession()
session.delegate = self
let signatureGenerator = SHSignatureGenerator()
try signatureGenerator.append(audioBuffer, at: AVAudioTime.seconds(forHostTime: 0))
let signature = signatureGenerator.signature()
session.match(signature)
}
func session(_ session: SHSession, didFind match: SHMatch) {
// Hurray you got the matching song
}
func session(_ session: SHSession, didNotFindMatchFor signature: SHSignature, error: Error?) {
// Ohh No.. couldn't find any matching
}
}
You need to add the following key in info.plist for permission to use microphone in order to record audio.
Key = Privacy - Microphone Usage Description and Value = To find song from hymn
Warning: You can test ShazhamKit only in device and not in Simulator.
MusicKit
Now you can play songs right from Apple Music in your app. You can integrate MusicKit framework in any of you iOS or iPadOS apps.
Not only that it now allow Apple Music to be integrated in Android app and Websites as well. I know you can’t believe that. Android is no more an enemy đ
However users need to have their their Apple Music subscription to enjoy this sdk.
Adding to it Apple also providing web api to consume Apple Music. Check out this apple developer link. Which has nothing to do with iOS 15
To use the MusicKit in iOS apps there is a key which needs to added to info.plist
Key: Privacy - Media Library Usage Description Value: To let user play music of their choice
There is a nice sample code provided by, I will dissect in future and write a simpler code but for now please refer this.
CoreLocationUI
To access user’s current location you can have a button, a specialized button and it’s look you won’t be able to change. Which means it will give user a familier ui where to provide their location when requested.
Like Apple Pay button which you can’t change, similarly this core location button
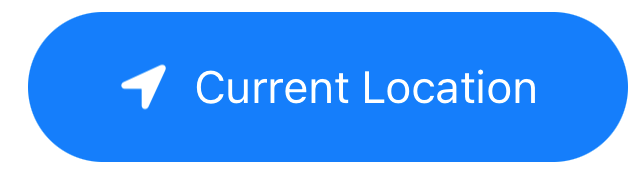
But the thing it’s a swift ui button called LocationButton
Though you slightly customize it but not too much.
LocationButton(.currentLocation) {
// Fetch location with Core Location.
}.symbolVariant(.fill).labelStyle(.titleAndIcon)
Fetching the location using CoreLocation remain same. You can refer this stack overflow link to get the location as usual.
Group Activity & Share Play
Group Activity gives user the feeling that they and their friends are in same room, watching a movie, drawing a cartoon or jamming on music.
It’s basically doing/watching something together, though actually everyone will consume the video from their own app or subscription. However it gives a fake feeling of living in the moment.
Let’s say while watching a movie together with your friend you found something very funny which you would like to show your friend by rewinding to last 10 secs, you can do it, and all of your friends will be able to see that same rewinded scene and laugh together.
It basically happens over FaceTime.
Group Activity session has to be created by following a protocol called GroupActivity
To integrate the Group Activity and SharePlay follow this link from Apple
Reference: https://developer.apple.com/ios/